Advanced Performance Optimization Techniques for Modern Web Applications
Iliya Timohin
2025-01-10
Picture this: You're browsing a website, and those precious seconds tick by as you wait for content to load. We've all been there, and let's be honest – waiting is the last thing anyone wants to do online. As developers, we're facing a reality where a single second of delay can cost thousands in lost revenue and send potential customers straight to our competitors. The bar for web performance optimization isn't just high – it's constantly rising. Let's dive into the cutting-edge website performance techniques that separate lightning-fast web applications from the rest of the pack, including strategies for improving Core Web Vitals. From sophisticated caching strategies to the latest in code splitting, we'll cover the advanced optimization methods that can transform your application's performance from a potential liability into a competitive advantage. This article will provide you with actionable insights and practical techniques to optimize your web applications for speed and user experience.
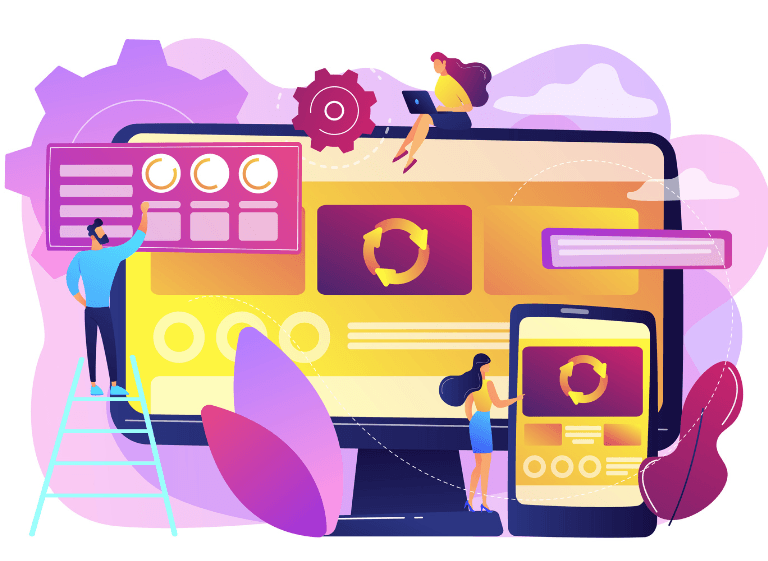
The Crucial Role of Web Performance Optimization in Modern Web Development
Web performance optimization plays a crucial role in modern web development, directly influencing key website metrics such as user engagement and conversion rates. Research by Google has consistently demonstrated the significant impact of website speed on user behavior. Even a few seconds of delay in page load time can drastically reduce conversions and increase bounce rates, causing potential customers to abandon their visit before even exploring your offerings. Google Research on Website Performance and User Behavior.
Core Web Vitals are user-centric metrics that reflect the real-world user experience of a website. Optimizing Core Web Vitals like Largest Contentful Paint (LCP optimization), First Input Delay (FID improvement), and Cumulative Layout Shift (CLS metrics) is critical for providing a seamless and enjoyable user experience. Improvements in these web performance metrics directly translate to business benefits, leading to better user retention, increased engagement, and higher conversion rates. Learn about Core Web Vitals.
Effective Caching Strategies for High-Traffic Web Applications
Caching strategies are essential for reducing server load and dramatically improving website performance. By efficiently storing website data, caching techniques minimize the need for repeated server requests, resulting in faster loading times and improved user experience. Several effective caching strategies exist:
- Browser Caching Techniques: Browser caching stores static resources, such as images, CSS stylesheets, and JavaScript files, directly on the user's browser. This reduces the need to fetch these resources from the server on subsequent visits, significantly improving website speed. Browser caching is controlled through HTTP headers like Cache-Control, Expires, ETag, and Last-Modified. A common example is setting Cache-Control: public, max-age=31536000 to cache a resource for a year. HTTP Caching.
- CDN Performance Optimization: Content Delivery Networks (CDNs) distribute website content across a network of geographically dispersed servers. This reduces latency and accelerates content delivery for users worldwide, regardless of their location. CDN performance is crucial for websites with a global audience.
- Server-Side Caching Strategies: Server-side caching utilizes in-memory data stores like Redis or Memcached to cache frequently accessed data, such as database queries and API responses. This significantly reduces database load and improves server response times, leading to faster website loading times. Effective cache invalidation strategies are essential for maintaining data consistency.
Optimizing Page Load with Image Lazy Loading Techniques
Image lazy loading is a powerful front-end performance optimization technique that defers the loading of offscreen images until they are about to become visible in the viewport. This significantly reduces initial page load time and improves perceived website performance. Several lazy loading techniques can be used:
- Intersection Observer API for Lazy Loading: The Intersection Observer API is a modern JavaScript API that efficiently detects when an element intersects with the viewport. This allows for precise control over when images are loaded, ensuring optimal performance.
const images = document.querySelectorAll('img[data-src]');
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
img.removeAttribute('data-src');
observer.unobserve(img);
}
});
});
images.forEach(image => {
observer.observe(image);
});
- Native Image Lazy Loading with loading="lazy": The loading="lazy" attribute provides a simple and efficient way to implement image lazy loading directly in HTML. This eliminates the need for JavaScript in basic cases.
<img src="placeholder.jpg" data-src="image.jpg" loading="lazy" alt="My Image">
It's important to avoid lazy loading images above the fold (within the initial viewport) as this can negatively impact initial rendering and LCP.
For simplified implementation of image lazy loading and advanced image optimization, Pinta offers solutions like Image Compress with Squeezeimg for CS-Cart and Image Convert Pro for OpenCart/PrestaShop. These solutions provide automated lazy loading, image compression, format conversion (WebP, AVIF), and CDN integration to maximize website speed and front-end performance.
JavaScript Code Splitting for Improved Website Loading Speed
JavaScript code splitting is a powerful technique for optimizing website loading speed by breaking down large JavaScript bundles into smaller, on-demand chunks. This reduces the amount of JavaScript that needs to be downloaded and parsed initially, leading to faster page load times and improved user experience. Module bundling tools like Webpack and Vite provide robust support for JavaScript code splitting through dynamic imports and other features. Code Splitting.
- Route-Based Code Splitting: This strategy splits code based on website routes, loading only the necessary JavaScript for the current page.
- Component-Based Code Splitting: This approach further optimizes loading efficiency by loading individual components only when they are required.
// Example of dynamic imports for JavaScript code splitting
async function loadComponent() {
const { MyComponent } = await import('./MyComponent');
// ... use MyComponent
}
Pinta's Image Compress with Squeezeimg for CS-Cart and Image Convert Pro for OpenCart/PrestaShop modules are built with efficient module bundling and code splitting strategies to minimize JavaScript bundle sizes and ensure optimal website performance.
Tree Shaking: Eliminating Dead Code from JavaScript Bundles
Tree shaking is a valuable technique for reducing JavaScript bundle sizes by removing unused code (dead code) during the build process. Static code analysis is used to identify and eliminate this dead code, resulting in smaller files and faster website loading times.
Advanced Image and Text Compression Techniques for Web Performance
Compression plays a vital role in minimizing website load time. Advanced compression algorithms like Brotli generally offer significantly better compression ratios compared to Gzip, resulting in smaller file sizes and faster download times. Other compression methods, such as Zopfli, can also be considered for further optimization. Image compression techniques, like those implemented in our modules, further reduce file sizes without significant quality loss.
Core Web Vitals Optimization: Key Metrics for User Experience
Optimizing Core Web Vitals is essential for providing a positive user experience and improving search engine rankings. Each metric requires specific optimization strategies:
- LCP (Largest Contentful Paint) Optimization: Focus on optimizing server response times, using efficient image formats (WebP, AVIF), and optimizing critical CSS delivery.
- FID (First Input Delay) Improvement: Minimize JavaScript execution time, break up long tasks, and optimize third-party scripts.
- CLS (Cumulative Layout Shift) Metrics Optimization: Use size attributes for images and ads, reserve space for dynamically loaded content, and avoid inserting content above existing content.
Utilizing tools like Chrome DevTools, PageSpeed Insights, and Search Console is crucial for monitoring and improving these user-centric metrics.
Website Performance Monitoring and Testing for Continuous Improvement
Continuous website performance monitoring and testing are vital for maintaining optimal website speed and identifying potential bottlenecks. Various tools, including Lighthouse, WebPageTest, GTmetrix, and Chrome User Experience Report (CrUX), can be used for performance analysis and website speed testing. Load testing and stress testing are essential for evaluating website stability and performance under high traffic conditions.
Integrating Advanced Optimization Techniques with Modern Front-End Frameworks
Modern front-end frameworks like React, Vue.js, and Next.js offer powerful features specifically designed for web performance optimization. Leveraging these features is crucial for building high-performing web applications.
- Server-Side Rendering (SSR): Server-side rendering renders web pages on the server and sends fully rendered HTML to the client. This dramatically reduces the time to first contentful paint (FCP) and improves SEO, as search engines can easily crawl and index the pre-rendered content. SSR is particularly beneficial for content-heavy websites and e-commerce platforms.
- Static Site Generation (SSG): Static site generation pre-renders web pages at build time, generating static HTML files that can be served directly from a CDN. This approach offers exceptional performance, especially for websites with static content, such as blogs and documentation sites.
- Framework-Specific Optimizations: Each framework offers its own set of performance optimization tools and techniques. For example, React offers features like code splitting with dynamic imports, memoization with React.memo, and virtualized lists for handling large datasets. Vue.js provides features like asynchronous components and lazy loading of routes. Next.js, a React framework, simplifies the implementation of SSR and SSG.
Choosing between SSR and SSG depends on the specific requirements of the web application. SSR is suitable for dynamic content that changes frequently, while SSG is ideal for static content that remains relatively constant.
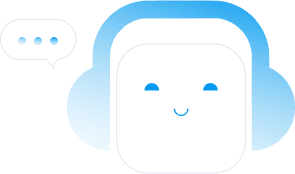
Leveraging Pinta's Expertise for Specialized Web Performance Challenges
While many web performance optimization techniques are well-documented and readily available, complex web development projects often encounter unique performance bottlenecks that require specialized expertise. At PintaWebWare, we have extensive experience in addressing these complex performance challenges, offering tailored solutions and expert consulting to enhance website speed and front-end performance. Our expertise encompasses:
- Comprehensive Core Web Vitals Optimization: We conduct in-depth analysis and implement targeted strategies to improve LCP, FID, and CLS, ensuring optimal user experience and search engine visibility.
- Advanced Compression Techniques and Image Optimization: We leverage advanced compression algorithms, including Brotli and Zopfli, and implement effective image optimization techniques, including format conversion to WebP and AVIF, to minimize file sizes without compromising quality. Our Image Compress with Squeezeimg for CS-Cart та Image Convert Pro for OpenCart/PrestaShop modules exemplify this expertise.
- Seamless Integration with Modern Front-End Frameworks: We have deep expertise in optimizing web applications built with modern front-end frameworks like React, Vue.js, and Next.js, leveraging their built-in performance features and implementing custom solutions to address specific performance bottlenecks.
- Performance Audits and Consulting: We provide comprehensive performance audits to identify areas for improvement and offer expert consulting to help businesses achieve their performance goals.
Contact us today to learn how Pinta can help you elevate your web application’s performance and deliver a superior user experience.